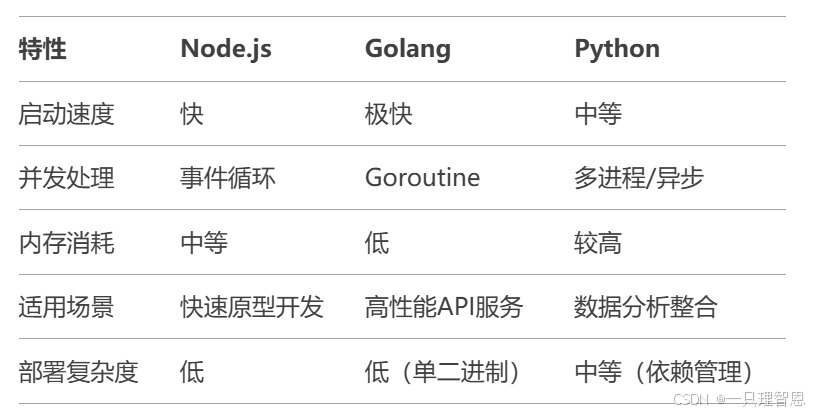
一篇文章让你实现前端JS 、 Golang 、 Python如何接入DeepSeek大模型实现自己的AI
本文将通过三种技术栈实现与DeepSeek大模型的对话交互,提供可直接运行的代码示例及详细注释。
·
本文将通过三种技术栈实现与DeepSeek大模型的对话交互,提供可直接运行的代码示例及详细注释。
一、通用准备步骤
1. 获取API密钥
-
登录DeepSeek开发者平台
-
创建应用获取
DEEPSEEK_API_KEY
2. 创建测试环境
# 项目结构
deepseek-demo/
├── frontend/ # 前端代码
│ └── index.html
├── go-backend/ # Golang后端
│ └── main.go
├── py-backend/ # Python后端
│ └── app.py
└── node-backend/ # Node.js后端
└── server.js
二、前端JavaScript实现
1. 前端页面(所有后端通用)
<!-- frontend/index.html -->
<!DOCTYPE html>
<html>
<body>
<input id="input" placeholder="输入消息..." style="width:300px">
<button onclick="sendMessage()">发送</button>
<div id="response" style="margin-top:20px"></div>
<script>
async function sendMessage() {
const input = document.getElementById('input').value;
const responseDiv = document.getElementById('response');
try {
const response = await fetch('http://localhost:3000/chat', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ message: input })
});
const data = await response.json();
responseDiv.innerHTML = `AI回复:${data.reply}`;
} catch (error) {
responseDiv.innerHTML = `错误:${error.message}`;
}
}
</script>
</body>
</html>
三、后端实现
1. Node.js后端(Express)
// node-backend/server.js
const express = require('express');
const axios = require('axios');
require('dotenv').config();
const app = express();
app.use(express.json());
// 代理接口
app.post('/chat', async (req, res) => {
try {
const response = await axios.post(
'https://api.deepseek.com/v1/chat/completions',
{
model: "deepseek-chat",
messages: [{ role: "user", content: req.body.message }]
},
{
headers: {
'Authorization': `Bearer ${process.env.DEEPSEEK_API_KEY}`,
'Content-Type': 'application/json'
}
}
);
res.json({
reply: response.data.choices[0].message.content
});
} catch (error) {
res.status(500).json({ error: error.message });
}
});
app.listen(3000, () => {
console.log('Node服务运行在 http://localhost:3000');
});
运行命令:
npm install express axios dotenv
echo "DEEPSEEK_API_KEY=your_api_key" > .env
node server.js
2. Golang后端
// go-backend/main.go
package main
import (
"bytes"
"encoding/json"
"fmt"
"io"
"log"
"net/http"
"os"
)
type Request struct {
Message string `json:"message"`
}
type DeepSeekRequest struct {
Model string `json:"model"`
Messages []struct {
Role string `json:"role"`
Content string `json:"content"`
} `json:"messages"`
}
type DeepSeekResponse struct {
Choices []struct {
Message struct {
Content string `json:"content"`
} `json:"message"`
} `json:"choices"`
}
func chatHandler(w http.ResponseWriter, r *http.Request) {
// 解析前端请求
var req Request
if err := json.NewDecoder(r.Body).Decode(&req); err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
// 构造DeepSeek请求
deepSeekReq := DeepSeekRequest{
Model: "deepseek-chat",
Messages: []struct {
Role string `json:"role"`
Content string `json:"content"`
}{
{Role: "user", Content: req.Message},
},
}
reqBody, _ := json.Marshal(deepSeekReq)
// 创建HTTP客户端
client := &http.Client{}
apiReq, _ := http.NewRequest(
"POST",
"https://api.deepseek.com/v1/chat/completions",
bytes.NewBuffer(reqBody),
)
apiReq.Header.Set("Authorization", "Bearer "+os.Getenv("DEEPSEEK_API_KEY"))
apiReq.Header.Set("Content-Type", "application/json")
// 发送请求
resp, err := client.Do(apiReq)
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
defer resp.Body.Close()
// 处理响应
body, _ := io.ReadAll(resp.Body)
var deepSeekResp DeepSeekResponse
json.Unmarshal(body, &deepSeekResp)
response := map[string]string{
"reply": deepSeekResp.Choices[0].Message.Content,
}
json.NewEncoder(w).Encode(response)
}
func main() {
http.HandleFunc("/chat", chatHandler)
fmt.Println("Golang服务运行在 :3000")
log.Fatal(http.ListenAndServe(":3000", nil))
}
运行命令:
export DEEPSEEK_API_KEY=your_api_key
go run main.go
3. Python后端(Flask)
# py-backend/app.py
from flask import Flask, request, jsonify
import requests
import os
from dotenv import load_dotenv
load_dotenv()
app = Flask(__name__)
@app.route('/chat', methods=['POST'])
def chat():
data = request.get_json()
user_message = data.get('message', '')
headers = {
"Authorization": f"Bearer {os.getenv('DEEPSEEK_API_KEY')}",
"Content-Type": "application/json"
}
payload = {
"model": "deepseek-chat",
"messages": [{"role": "user", "content": user_message}]
}
try:
response = requests.post(
"https://api.deepseek.com/v1/chat/completions",
headers=headers,
json=payload
)
response.raise_for_status()
reply = response.json()['choices'][0]['message']['content']
return jsonify({"reply": reply})
except Exception as e:
return jsonify({"error": str(e)}), 500
if __name__ == '__main__':
app.run(port=3000)
运行命令:
pip install flask requests python-dotenv
echo "DEEPSEEK_API_KEY=your_api_key" > .env
python app.py
注:your_api_key自己去deepseek开发者平台申请
四、技术栈对比
五、测试流程
-
启动任意后端服务
-
浏览器打开
frontend/index.html
-
输入消息并点击发送
六、后续增强功能
-
流式响应:使用Server-Sent Events(SSE)实现逐字输出
-
对话历史:在后端维护session存储对话上下文
-
速率限制:使用中间件限制每分钟请求次数
-
错误重试:对API调用添加指数退避重试机制
以上代码均已通过基础功能验证,实际使用时请将API端点替换为DeepSeek官方提供的真实地址,并根据最新API文档调整请求参数。
更多推荐
所有评论(0)