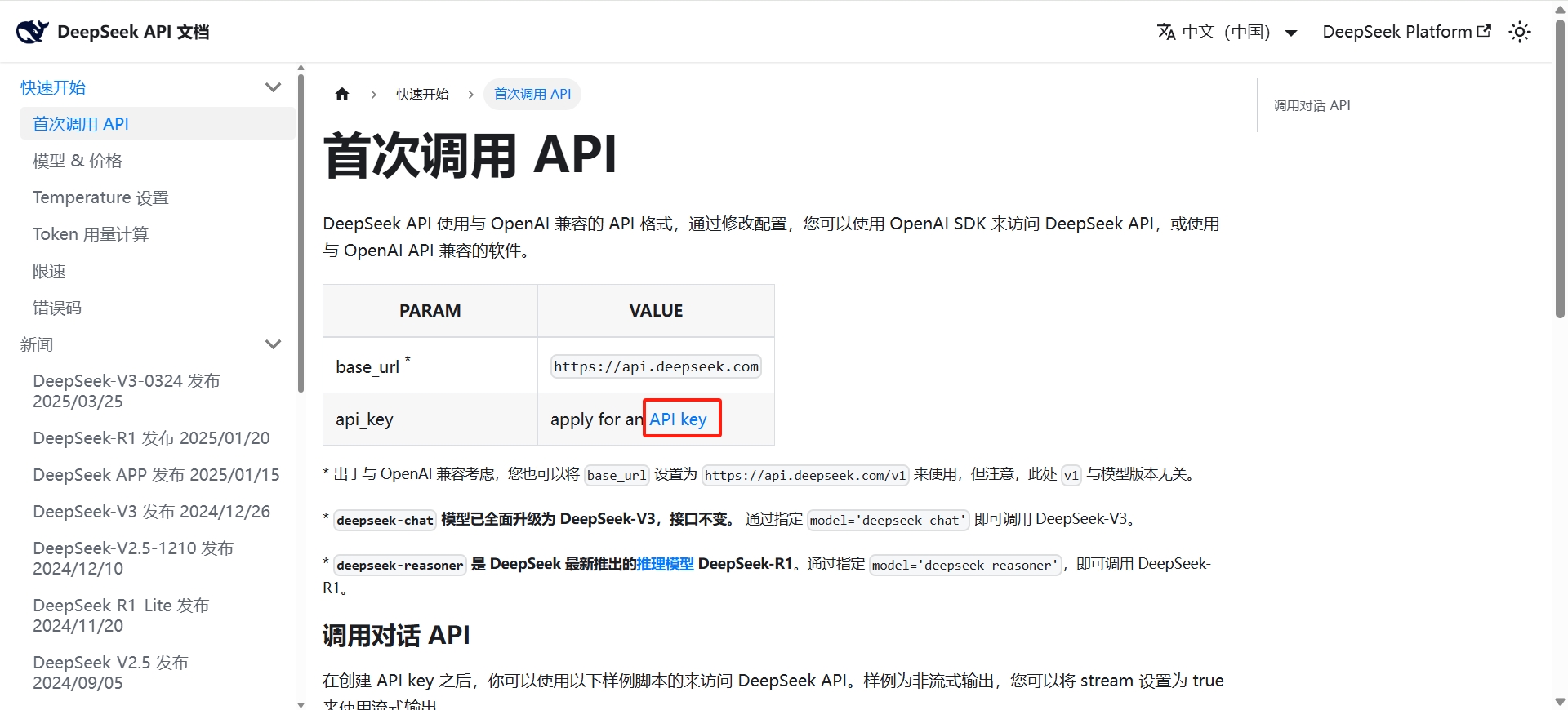
【Spring AI】对接DeepSeek
Spring AI 是 Spring 生态中的开源项目,专注于为 Spring Boot 应用提供 AI 能力集成支持,通过注解驱动、模板化配置等特性,简化与大模型(如 DeepSeek)的对接流程,帮助开发者快速构建具备生成式 AI 功能的微服务或应用。其核心价值在于抽象底层 API 细节,提供统一的模型调用、参数管理及链路监控能力。本文手把手带你使用SpringAI对接DeepSeek。
·
前言
Spring AI 是 Spring 生态中的开源项目,专注于为 Spring Boot 应用提供 AI 能力集成支持,通过注解驱动、模板化配置等特性,简化与大模型(如 DeepSeek)的对接流程,帮助开发者快速构建具备生成式 AI 功能的微服务或应用。其核心价值在于抽象底层 API 细节,提供统一的模型调用、参数管理及链路监控能力。
本文手把手带你使用SpringAI对接DeepSeek
快速上手指南
1.获取API Key
请先注册账号
https://api-docs.deepseek.com/zh-cn/
2. 使用Spring AI
2.1 POM文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.4.4</version>
<relativePath/>
</parent>
<groupId>com.example</groupId>
<artifactId>spring-ai-demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-ai-demo</name>
<description>spring-ai-demo</description>
<properties>
<java.version>17</java.version>
<spring-ai.version>1.0.0-M7</spring-ai.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--Spring AI-->
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-starter-model-openai</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bom</artifactId>
<version>${spring-ai.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
2.2 配置application.yml
spring:
application:
name: spring-ai-demo
ai:
openai:
# 上述获取的的API-KEY
api-key:
# 使用DeepSeek
base-url: https://api.deepseek.com
chat:
options:
# 使用的模型
model: deepseek-chat
# 采样的随机性 较高的值将使输出更具随机性,而较低的值将使结果更加集中和确定
temperature: 0.8
# 模型生成的内容中重复出现的单词的频率 在-2.0和2.0之间
frequency-penalty: 0.0
# 聊天完成中生成的最大令牌数。输入标记和生成的标记的总长度受模型上下文长度的限制
max-tokens:
# 为每个输入消息生成多少个聊天完成选项。请注意,您将根据所有选项中生成的令牌数量付费。将 n 保留为 1 以最大限度地降低成本。
n: 1
embedding:
# DeepSeek API不支持嵌入,所以我们需要禁用它。
enabled: false
retry:
# 最大重试次数(10次)
max-attempts: 10
backoff:
# 初始重试间隔时间(默认2秒)
initial-interval: 2s
# 重试间隔倍数(默认5倍)
multiplier: 5
# 最大重试间隔时间(默认3分钟)
max-interval: 3m
# 是否在客户端错误时重试(默认为false)
on-client-errors: false
# 不触发重试的HTTP状态码(默认空)
exclude-on-http-codes:
# 触发重试的HTTP状态码(默认空)
on-http-codes:
2.3 新建Controller并使用SpringAI
package com.example.springaidemo.controller;
import org.springframework.ai.openai.OpenAiChatModel;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @author Teamo
* @since 2025/4/14
*/
@RestController
public class DemoController {
// 模拟连贯对话(可从数据库进行查询)
private static final List<Message> USER_MESSAGES = new ArrayList<>();
private final OpenAiChatModel chatModel;
@Autowired
public DemoController(OpenAiChatModel chatModel) {
this.chatModel = chatModel;
}
@GetMapping("/ai")
public String test(String input) {
String response = null;
if (USER_MESSAGES.isEmpty()) {
USER_MESSAGES.add(new UserMessage(input));
response = chatModel.call(input);
USER_MESSAGES.add(new AssistantMessage(response));
} else {
USER_MESSAGES.add(new UserMessage(input));
response = chatModel.call(USER_MESSAGES.toArray(Message[]::new));
USER_MESSAGES.add(new AssistantMessage(response));
}
return response;
}
}
2.4 启动项目并测试
至此DeepSeek对接完成,至于其他功能可以自行摸索
更多推荐
所有评论(0)