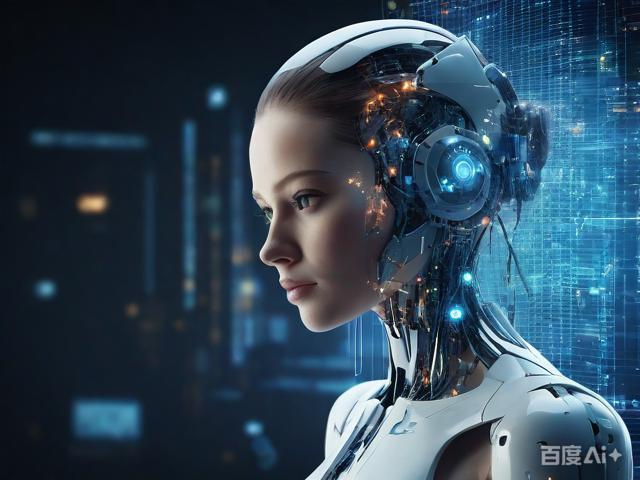
JAVA接入DeepSeek R1满血版,附带源代码以及注释,还有接入方法,教程
本文主要介绍了JAVA集成本地部署的DeepSeek的图文教程,包含配置环境变量及下载DeepSeek-R1模型并启动,具有一定的参考价值,感兴趣的可以了解一下,以下为基于 Java 技术栈快速实现 DeepSeek 大模型调用的综合方案,整合了多篇技术实践文档的核心内容。
·
JAVA接入DeepSeek R1满血版,附带源代码以及注释,还有接入方法,教程
回答
本文主要介绍了JAVA集成本地部署的DeepSeek的图文教程,包含配置环境变量及下载DeepSeek-R1模型并启动,具有一定的参考价值,感兴趣的可以了解一下,以下为基于 Java 技术栈快速实现 DeepSeek 大模型调用的综合方案,整合了多篇技术实践文档的核心内容。
Java集成DeepSeek R1模型教程
一、开发环境准备
Java
// 使用Maven添加依赖(pom.xml)
<dependencies>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.10.0</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.10.1</version>
</dependency>
</dependencies>
二、核心接入代码实现
Java
import okhttp3.*;
import com.google.gson.Gson;
import java.io.IOException;
public class DeepSeekR1Client {
private static final String API_URL = "https://api.deepseek.com/v1/r1/predict";
private final OkHttpClient client = new OkHttpClient();
private final Gson gson = new Gson();
// 请求数据结构
private static class RequestBody {
String model = "r1-8b";
String prompt;
int max_tokens = 200;
double temperature = 0.7;
}
// 响应数据结构
private static class ResponseBody {
Prediction[] predictions;
static class Prediction {
String text;
double probability;
}
}
/**
* 发送请求到DeepSeek R1模型
* @param apiKey 认证密钥
* @param prompt 输入文本
* @return 模型生成的响应
*/
public String generateText(String apiKey, String prompt) throws IOException {
// 构建请求体
RequestBody requestBody = new RequestBody();
requestBody.prompt = prompt;
String json = gson.toJson(requestBody);
// 创建HTTP请求
Request request = new Request.Builder()
.url(API_URL)
.addHeader("Authorization", "Bearer " + apiKey)
.addHeader("Content-Type", "application/json")
.post(RequestBody.create(json, MediaType.get("application/json")))
.build();
// 执行请求并解析响应
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
ResponseBody body = gson.fromJson(response.body().charStream(), ResponseBody.class);
return body.predictions[0].text;
}
}
}
三、代码使用示例
Java
public class Main {
public static void main(String[] args) {
DeepSeekR1Client client = new DeepSeekR1Client();
try {
String result = client.generateText(
"your_api_key_here",
"解释量子计算的基本原理"
);
System.out.println("模型响应:" + result);
} catch (IOException e) {
e.printStackTrace();
}
}
}
四、关键参数说明
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
model | String | r1-8b | 模型版本标识符 |
max_tokens | int | 200 | 最大输出token数 |
temperature | double | 0.7 | 生成多样性控制(0-1) |
五、注意事项
- API密钥需从DeepSeek平台获取1
- 建议添加重试机制处理网络波动
- 注意控制请求频率避免触发限流
- 生产环境应使用连接池配置
六、思维导图
七、创建deepseek账户
deepseek网址:https://www.deepseek.com/
创建key
记住生成的key,在其他地方保存,一会要用。
八、API代码部分
引入库
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.3</version>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.12.0</version>
</dependency>
代码如下:
package com.example.demo;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class DeepSeekAppR extends JFrame {
private JTextField inputField;
private JTextArea outputArea;
public DeepSeekAppR() {
// 设置窗口标题
setTitle("DeepSeek Chat");
// 设置窗口大小
setSize(600, 400);
// 设置关闭窗口时的操作
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 设置布局管理器
setLayout(new BorderLayout());
// 创建输入框
inputField = new JTextField();
// 将输入框添加到窗口底部
add(inputField, BorderLayout.SOUTH);
// 创建文本区域用于显示输出
outputArea = new JTextArea();
outputArea.setEditable(false);
JScrollPane scrollPane = new JScrollPane(outputArea);
// 将滚动面板添加到窗口中心
add(scrollPane, BorderLayout.CENTER);
// 为输入框添加事件监听器
inputField.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String input = inputField.getText();
if (!input.isEmpty()) {
// 调用 DeepSeek API
String response1= "";
String response = callDeepSeekAPI(input);
System.out.println(response);
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode rootNode = objectMapper.readTree(response);
JsonNode choicesNode = rootNode.get("choices");
if (choicesNode.isArray() && choicesNode.size() > 0) {
JsonNode firstChoice = choicesNode.get(0);
JsonNode messageNode = firstChoice.get("message");
response = messageNode.get("content").asText();
response1 = messageNode.get("reasoning_content").asText();
System.out.println(response1);
System.out.println(response);
}
} catch (IOException r) {
r.printStackTrace();
}
// 在输出区域显示响应
outputArea.append("You: " + input + "\n");
outputArea.append("DeepSeek思考: " + response1 + "\n\n");
outputArea.append("DeepSeek: " + response + "\n\n");
// 清空输入框
inputField.setText("");
}
}
});
}
private String callDeepSeekAPI(String input) {
try {
// 替换为实际的 DeepSeek API 端点
URL url = new URL("https://api.deepseek.com/v1/chat/completions");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
// 替换为你的 DeepSeek API 密钥
connection.setRequestProperty("Authorization", "Bearer 你的key");
connection.setDoOutput(true);
// 构建请求体
String requestBody = "{\"model\": \"deepseek-reasoner\",\"messages\": [{\"role\": \"user\", \"content\": \"" + input + "\"}]}";
System.out.println(requestBody);
OutputStream outputStream = connection.getOutputStream();
outputStream.write(requestBody.getBytes());
outputStream.flush();
outputStream.close();
// 获取响应
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
// 解析 JSON 响应,这里简单返回原始响应
return response.toString();
} else {
return "Error: " + responseCode;
}
} catch (IOException ex) {
ex.printStackTrace();
return "Error: " + ex.getMessage();
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
DeepSeekAppR app = new DeepSeekAppR();
app.setVisible(true);
}
});
}
}
此处的key是上面生成的key。
部分截图:
HTML部分代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>智能 AI</title>
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css" rel="external nofollow" >
<link rel="stylesheet" href="/css/self.css" rel="external nofollow" >
</head>
<body>
<div id="app">
<div class="chat-container">
<div v-if="showTitle" class="welcome-title">你好!我是CloseAI,有什么可以帮助你的吗?</div>
<div class="chat-messages">
<transition-group name="list">
<div v-for="message in messages" :key="message.id" class="message-wrapper"
:class="{'user-message-wrapper': message.sender === 'user', 'ai-message-wrapper': message.sender === 'ai'}">
<img :src="message.sender === 'user' ? '/images/user.png' : '/images/ai.png'" class="avatar"/>
<div class="message"
:class="{'user-message': message.sender === 'user', 'ai-message': message.sender === 'ai'}">
<span v-if="message.sender === 'user'">{{ message.text }}</span>
<div v-else v-html="message.formattedText || message.text"></div>
<div v-if="message.sender === 'ai' && message.isLoading" class="loading-icon"></div>
<!-- 调整停止按钮容器位置 -->
<div v-if="(isThinking || animationFrameId) && message.sender === 'ai' && message.isActive"
class="stop-container">
<button @click="stopThinking" class="stop-btn" title="停止响应">
<svg width="24" height="24" viewBox="0 0 24 24">
<rect x="6" y="4" width="4" height="16" rx="1"/>
<rect x="14" y="4" width="4" height="16" rx="1"/>
</svg>
</button>
</div>
</div>
</div>
</transition-group>
</div>
<div class="chat-input">
<input type="text" v-model="userInput" @keyup.enter="sendMessage" placeholder="给 CloseAI 发送消息..."/>
<button @click="sendMessage">Send</button>
</div>
</div>
</div>
<script src="/js/vue.js"></script>
<script src="/js/axios.min.js"></script>
<script src="/js/method.js"></script>
<script src="https://cdn.jsdelivr.net/npm/marked/marked.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/dompurify/3.0.5/purify.min.js"></script>
<script>
function debounce(func, wait) {
let timeout;
return function (...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), wait);
};
}
new Vue({
el: '#app',
data: {
userInput: '',
messages: [],
showTitle: true, // 添加一个新属性来控制标题的显示
performanceConfig: {
maxMessageLength: 1000, // 超过该长度启用优化
chunkSize: window.innerWidth < 768 ? 3 : 5 // 响应式分段
},
// 新增控制变量
isThinking: false,
currentController: null, // 请求中止控制器
animationFrameId: null // 动画帧ID
},
methods: {
sendMessage() {
if (this.userInput.trim() !== '') {
this.showTitle = false; // 用户开始提问时隐藏标题
const userMessage = {
id: Date.now(),
sender: 'user',
text: this.userInput
};
this.messages.push(userMessage);
this.userInput = '';
// 停用所有旧消息
this.messages.forEach(msg => {
if (msg.sender === 'ai') {
this.$set(msg, 'isActive', false);
}
});
const aiMessage = {
id: Date.now() + 1,
sender: 'ai',
text: '思考中 ',
isLoading: true,
isThinking: true, // 新增状态字段
isActive: true // 新增激活状态字段
};
this.messages.push(aiMessage);
// 在请求前重置状态
this.isThinking = true;
// 使用 axios 的取消令牌
const controller = new AbortController();
this.currentController = controller;
axios.get('/deepSeek/chat?question=' + userMessage.text, {
signal: controller.signal
}).then(response => {
aiMessage.isLoading = false;
aiMessage.text = ''
aiMessage.rawContent = response.data; // 新增原始内容存储
let index = 0;
let chunk = '';
const chunkSize = this.performanceConfig.chunkSize;
let lastUpdate = 0;
let lastFullRender = 0;
const processChunk = () => {
// 分块处理逻辑
const remaining = response.data.length - index;
const step = remaining > chunkSize * 5 ? chunkSize * 5 : 1;
chunk += response.data.slice(index, index + step);
index += step;
// 增量更新文本
aiMessage.text = chunk;
this.safeParseMarkdown(aiMessage, true); // 实时解析
this.scrollToBottom();
};
const animate = (timestamp) => {
// 时间片控制逻辑
if (timestamp - lastUpdate > 16) { // 约60fps
processChunk();
lastUpdate = timestamp;
}
// 完整渲染控制(每100ms强制更新一次)
if (timestamp - lastFullRender > 100) {
this.$forceUpdate();
lastFullRender = timestamp;
}
if (timestamp - lastUpdate > 20) {
const remainingChars = response.data.length - index;
const step = remainingChars > chunkSize ? chunkSize : 1;
aiMessage.text += response.data.substr(index, step);
index += step;
this.safeParseMarkdown(aiMessage);
this.scrollToBottom();
lastUpdate = timestamp;
}
if (index < response.data.length) {
this.animationFrameId = requestAnimationFrame(animate);
} else {
this.$set(aiMessage, 'isActive', false); // 关闭激活状态
this.$set(aiMessage, 'formattedText',
DOMPurify.sanitize(marked.parse(aiMessage.text))
);
this.animationFrameId = null; // 新增重置
this.isThinking = false; // 在此处更新状态
this.finalizeMessage(aiMessage);
}
};
// 修改动画启动方式
this.animationFrameId = requestAnimationFrame(animate);
}).catch(error => {
if (error.name !== 'CanceledError') {
console.error('Error:', error);
}
}).finally(() => {
this.animationFrameId = null;
// 新增消息状态更新
this.messages.forEach(msg => {
if (msg.sender === 'ai') {
msg.isLoading = false;
msg.isThinking = false;
}
});
});
}
},
// 新增滚动方法
scrollToBottom() {
const container = this.$el.querySelector('.chat-messages');
container.scrollTop = container.scrollHeight;
},
// 优化的Markdown解析方法
safeParseMarkdown: debounce(function (aiMessage, immediate = false) {
if (immediate) {
// // 快速解析基础格式(粗体、斜体等)
// const quickFormatted = aiMessage.text
// .replace(/\*\*(.*?)\*\*/g, '<strong>$1</strong>')
// .replace(/\*(.*?)\*/g, '<em>$1</em>');
// 完整Markdown解析
this.$set(aiMessage, 'formattedText',
DOMPurify.sanitize(marked.parse(aiMessage.text))
);
} else {
// 完整Markdown解析
this.$set(aiMessage, 'formattedText',
DOMPurify.sanitize(marked.parse(aiMessage.text))
);
}
}, 50), // 完整解析保持50ms防抖
stopThinking() {
// 只停止当前活动消息
const activeMessage = this.messages.find(msg =>
msg.sender === 'ai' && msg.isActive
);
if (activeMessage) {
// 更新消息状态
this.$set(activeMessage, 'text', activeMessage.text + '\n(响应已停止)');
this.$set(activeMessage, 'isLoading', false);
this.$set(activeMessage, 'isActive', false);
this.safeParseMarkdown(activeMessage);
}
// 取消网络请求
if (this.currentController) {
this.currentController.abort()
}
// 停止打印渲染
if (this.animationFrameId) {
cancelAnimationFrame(this.animationFrameId);
this.animationFrameId = null;
}
// 更新所有相关消息状态
this.messages.forEach(msg => {
if (msg.sender === 'ai' && (msg.isLoading || msg.isThinking)) {
msg.text += '\n(响应已停止)';
msg.isLoading = false;
msg.isThinking = false;
this.safeParseMarkdown(msg);
}
});
this.isThinking = false;
this.animationFrameId = null; // 确保重置
},
// 新增最终处理函数
finalizeMessage(aiMessage) {
this.$set(aiMessage, 'formattedText',
DOMPurify.sanitize(marked.parse(aiMessage.text))
);
this.$set(aiMessage, 'isActive', false);
this.animationFrameId = null;
this.isThinking = false;
this.$forceUpdate();
},
}
});
</script>
</body>
</html>
css样式:
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
.chat-container {
width: 100%;
/*max-width: 800px;*/
background-color: #fff;
border-radius: 12px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
overflow: hidden;
display: flex;
flex-direction: column;
}
.welcome-title {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
text-align: center;
font-size: 24px;
font-weight: bold;
}
/* 新增停止按钮样式 */
.stop-btn {
background-color: #ff4d4f;
color: white;
margin-left: 8px;
transition: all 0.3s;
}
.stop-btn:hover {
background-color: #f5f7fa;
}
.avatar {
width: 40px;
height: 40px;
border-radius: 50%;
margin-right: 10px;
vertical-align: middle;
}
.chat-messages {
/*flex: 1;*/
overflow-y: auto;
padding: 20px;
/*display: flex;*/
flex-direction: column-reverse;
height: 70vh;
width: 500px;
scrollbar-width: none;
scroll-behavior: smooth; /* 平滑滚动效果 */
contain: strict; /* 限制浏览器重排范围 */
transform: translateZ(0);
}
.message {
margin-bottom: 15px;
padding: 12px;
border-radius: 12px;
max-width: 70%;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
transition: all 0.3s ease;
/*align-self: flex-end; !* 默认消息靠右对齐 *!*/
display: table;
}
.message-wrapper {
display: flex;
max-width: 80%;
margin: 10px;
align-items: flex-start;
will-change: transform, opacity; /* 启用GPU加速 */
}
/*.user-message-wrapper {*/
/* justify-content: flex-end;*/
/*}*/
.ai-message-wrapper {
justify-content: flex-start;
position: relative; /* 为绝对定位的停止按钮提供参考系 */
margin-bottom: 50px; /* 给停止按钮留出空间 */
}
.avatar {
width: 40px;
height: 40px;
border-radius: 50%;
margin-right: 10px;
vertical-align: middle;
}
.message {
margin-bottom: 0;
}
.user-message {
background-color: #007bff;
color: #fff;
margin-left: auto; /* 用户消息靠右对齐 */
}
.ai-message {
background-color: #f0f2f5; /* AI消息背景色 */
margin-right: auto; /* 消息内容靠左 */
position: relative; /* 添加相对定位 */
padding-bottom: 40px; /* 为按钮预留空间 */
}
/* 在self.css中添加 */
.user-message-wrapper {
flex-direction: row-reverse; /* 反转排列方向 */
margin-left: auto; /* 让用户消息靠右 */
}
.user-message-wrapper .avatar {
margin: 0 0 0 15px; /* 调整头像右边距 */
}
.chat-input {
display: flex;
align-items: center;
padding: 15px;
background-color: #f8f9fa;
}
.chat-input input {
flex: 1;
padding: 12px;
border: 1px solid #ccc;
border-radius: 6px;
margin-right: 15px;
transition: border-color 0.3s ease;
}
.chat-input input:focus {
border-color: #007bff;
}
.chat-input button {
padding: 12px 24px;
border: none;
border-radius: 6px;
background-color: #007bff;
color: #fff;
cursor: pointer;
transition: background-color 0.3s ease;
}
.message {
display: flex;
align-items: center;
}
/* 调整停止按钮定位 */
.stop-container {
position: absolute;
bottom: 10px;
right: 10px;
z-index: 10;
}
.stop-btn {
background: #ff4757;
border: none;
border-radius: 50%;
width: 32px;
height: 32px;
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
box-shadow: 0 2px 5px rgba(0,0,0,0.2);
transition: transform 0.2s;
}
.stop-btn:hover {
transform: scale(1.1);
}
.stop-btn svg {
fill: white;
width: 16px;
height: 16px;
}
.ai-message pre {
background: #f5f7fa;
padding: 15px;
border-radius: 6px;
overflow-x: auto;
}
.ai-message code {
font-family: 'Courier New', monospace;
font-size: 14px;
}
.ai-message table {
border-collapse: collapse;
margin: 10px 0;
}
.ai-message td, .ai-message th {
border: 1px solid #ddd;
padding: 8px;
}
.loading-icon {
width: 20px;
height: 20px;
border: 2px solid #ccc;
border-top-color: #007bff;
border-radius: 50%;
/*animation: spin 1s linear infinite;*/
margin-left: 10px;
/* 替换为CSS动画实现的加载效果 */
/*background: linear-gradient(90deg, #eee 25%, #ddd 50%, #eee 75%);*/
animation: spin 1s infinite linear;
}
@keyframes spin {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
.chat-input button:hover {
background-color: #0056b3;
}
.list-enter-active, .list-leave-active {
transition: all 0.5s ease;
}
.list-enter, .list-leave-to /* .list-leave-active in <2.1.8 */ {
opacity: 0;
transform: translateY(30px);
}
九、示例演示及源码下载
实例演示:
演示地址:点击前往
源码下载:
下载地址: 点击前往
—— END ——
更多推荐
所有评论(0)