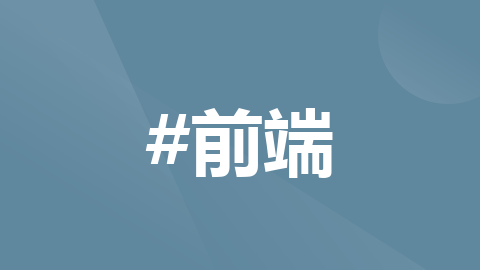
通过API和Web界面查询Dify+Ollama+DeepSeek
参考文档:</strong><ul><strong>回答:
·
通过API和Web界面查询Dify+Ollama+DeepSeek知识库的具体实现
一、通过Web界面查询
-
访问Dify Web界面:
- 默认地址:
http://localhost:3000
(如果在本地部署) - 登录后选择你创建的知识库应用
- 默认地址:
-
直接问答:
- 在聊天界面输入问题,系统会自动从知识库中检索相关信息并生成回答
二、通过API查询知识库
1. 获取API密钥
在Dify的"设置" > "API密钥"中创建一个新的API密钥
2. Python代码示例
import requests
import json
# 配置参数
API_KEY = "your-dify-api-key" # 替换为你的Dify API密钥
APP_ID = "your-app-id" # 替换为你的应用ID
API_URL = "http://localhost/v1/chat-messages" # 本地部署URL
# 如果是云服务可能是: "https://api.dify.ai/v1/chat-messages"
def query_knowledge_base(question):
headers = {
"Authorization": f"Bearer {API_KEY}",
"Content-Type": "application/json"
}
payload = {
"inputs": {},
"query": question,
"response_mode": "blocking", # 也可以是"streaming"流式响应
"user": "api-user" # 可自定义用户标识
}
response = requests.post(
f"{API_URL}/{APP_ID}",
headers=headers,
data=json.dumps(payload)
)
if response.status_code == 200:
return response.json()
else:
raise Exception(f"API请求失败: {response.status_code}, {response.text}")
# 使用示例
if __name__ == "__main__":
question = "你们公司的主要产品是什么?" # 替换为你的问题
try:
result = query_knowledge_base(question)
print("回答内容:")
print(result['answer'])
print("\n引用的知识文档:")
for doc in result.get('retriever_resources', []):
print(f"- {doc['content'][:100]}...") # 只打印前100字符
except Exception as e:
print(f"查询出错: {str(e)}")
3. 流式响应API调用
如果需要流式响应(适合长回答):
def stream_knowledge_base(question):
headers = {
"Authorization": f"Bearer {API_KEY}",
"Content-Type": "application/json",
"Accept": "text/event-stream" # 重要:请求流式响应
}
payload = {
"inputs": {},
"query": question,
"response_mode": "streaming",
"user": "api-user"
}
with requests.post(
f"{API_URL}/{APP_ID}",
headers=headers,
data=json.dumps(payload),
stream=True
) as response:
if response.status_code != 200:
raise Exception(f"API请求失败: {response.status_code}, {response.text}")
print("开始接收流式响应:")
for line in response.iter_lines():
if line:
decoded_line = line.decode('utf-8')
if decoded_line.startswith('data:'):
data = json.loads(decoded_line[5:])
if 'event' in data and data['event'] == 'message':
print(data['answer'], end='', flush=True)
print("\n回答完成")
# 使用示例
stream_knowledge_base("请详细说明你们的产品特点")
三、使用cURL命令查询
curl -X POST "http://localhost/v1/chat-messages/your-app-id" \
-H "Authorization: Bearer your-dify-api-key" \
-H "Content-Type: application/json" \
-d '{
"inputs": {},
"query": "你们公司的主要产品是什么?",
"response_mode": "blocking",
"user": "curl-user"
}'
四、前端集成示例(HTML+JavaScript)
<!DOCTYPE html>
<html>
<head>
<title>知识库查询</title>
<style>
#chat-container { width: 600px; margin: 0 auto; }
#response { border: 1px solid #ccc; min-height: 200px; padding: 10px; margin: 10px 0; }
</style>
</head>
<body>
<div id="chat-container">
<h2>知识库查询</h2>
<input type="text" id="question" placeholder="输入你的问题..." style="width: 80%">
<button onclick="queryKnowledge()">提问</button>
<div id="response"></div>
</div>
<script>
const API_KEY = "your-dify-api-key";
const APP_ID = "your-app-id";
const API_URL = "http://localhost/v1/chat-messages";
function queryKnowledge() {
const question = document.getElementById('question').value;
const responseDiv = document.getElementById('response');
responseDiv.innerHTML = "思考中...";
fetch(`${API_URL}/${APP_ID}`, {
method: 'POST',
headers: {
'Authorization': `Bearer ${API_KEY}`,
'Content-Type': 'application/json'
},
body: JSON.stringify({
inputs: {},
query: question,
response_mode: "blocking",
user: "web-user"
})
})
.then(response => response.json())
.then(data => {
responseDiv.innerHTML = `<strong>回答:</strong><p>${data.answer}</p>`;
if(data.retriever_resources && data.retriever_resources.length > 0) {
responseDiv.innerHTML += `<strong>参考文档:</strong><ul>`;
data.retriever_resources.forEach(doc => {
responseDiv.innerHTML += `<li>${doc.content.substring(0, 150)}...</li>`;
});
responseDiv.innerHTML += `</ul>`;
}
})
.catch(error => {
responseDiv.innerHTML = `查询出错: ${error.message}`;
});
}
</script>
</body>
</html>
五、API响应示例
成功的API响应会返回类似以下JSON结构:
{
"answer": "我们公司的主要产品是AI开发平台,提供自然语言处理、知识库管理和模型部署等功能...",
"retriever_resources": [
{
"content": "公司产品手册2023版...我们的旗舰产品是Dify AI开发平台...",
"file_name": "product_manual.pdf",
"page_number": 5
}
],
"metadata": {
"usage": {
"prompt_tokens": 45,
"completion_tokens": 128,
"total_tokens": 173
},
"model": "deepseek-llm:7b",
"timestamp": 1698765432
}
}
六、高级查询参数
你可以在API请求中添加更多参数来优化查询:
payload = {
"inputs": {},
"query": question,
"response_mode": "blocking",
"user": "advanced-user",
"conversation_id": "12345", # 用于多轮对话保持上下文
"temperature": 0.7, # 控制创造性(0-1)
"top_k": 50, # 从top_k个最可能的token中选择
"max_tokens": 500, # 限制响应长度
"retrieve_config": {
"top_k": 3, # 从知识库返回的最相关文档数
"score_threshold": 0.7 # 相关性阈值
}
}
以上代码提供了从基础到高级的各种方式来查询你的本地知识库系统。根据你的具体需求选择合适的方式集成到你的应用中。
更多推荐
所有评论(0)