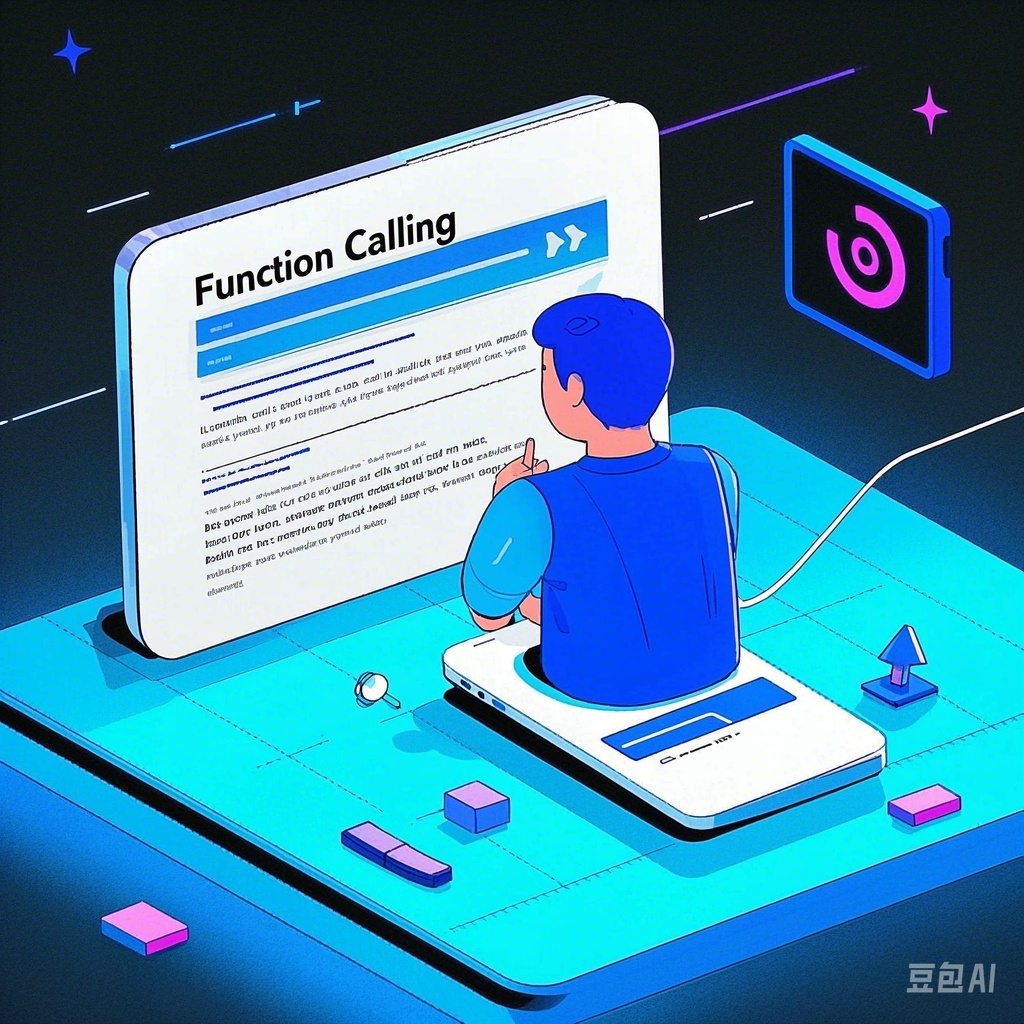
使用 DeepSeek 调用 Function Calling:从入门到实践
Function Calling 通过让AI模型无缝调用外部工具或服务,扩展其能力边界,实现从意图识别到执行落地的闭环,将大语言模型转化为可编程的智能执行中枢。
·
Fucntion Calling
1. 前言
- Function Calling 简介:
- 什么是 Function Calling?
- 为什么需要 Function Calling?
- DeepSeek 对 Function Calling 的支持。
- 目标读者:
- 对 AI 和自然语言处理感兴趣的程序员。
- 希望了解如何将 Function Calling 集成到应用中的开发者。
2. Function Calling 基础
- 什么是 Function Calling:
- 定义:Function Calling 是让 AI 模型调用预定义函数的能力。
- 示例:用户输入“现在时间”,AI 调用时间查询函数并返回结果。
- Function Calling 的优势:
- 扩展 AI 模型的能力,使其能够执行具体任务。
- 提高交互的灵活性和实用性。
- DeepSeek 的 Function Calling 支持:
- 支持的模型和功能。
- 与其他 API 的对比。
3. 准备工作
环境配置
环境配置请参照接入DeepSeek API,看这一篇就够了,超详细
安装依赖
确保你已经安装了openai sdk
pip install openai
4. Function Calling 的实现
4.1 定义函数
- 函数的作用:
- 示例:查询当前时间、查询天气、计算数学表达式、调用外部 API 等。
- 函数的格式:
- 函数名称、描述、参数列表。
- 示例代码:
def get_current_time(): current_datetime = datetime.now() formatted_time = current_datetime.strftime('%Y-%m-%d %H:%M:%S') return f"现在是 {formatted_time}"
4.2 配置 Function Calling
- 定义函数描述:
- 使用 JSON 格式描述函数,供 AI 模型识别。
- 示例代码:
functions = [ { "type": "function", "function": { "name": "get_current_time", "description": "当你想知道现在的时间时非常有用。", } }, ]
4.3 调用 DeepSeek API
- 发送请求:
- 将用户输入和函数描述发送到 DeepSeek API。
- 处理响应:
- 解析 API 返回的 JSON 数据,判断是否需要调用函数。
- 示例代码:
def chat_completion_request(messages, tools): print(f"正在像LLM发起API请求...") completion = client.chat.completions.create( model="deepseek-chat", # 此处以deepseek-chat为例 messages=messages, tools=tools ) print("返回对象:") print(completion.choices[0].message.model_dump_json()) print("\n") return completion
4.4 执行函数并返回结果
- 解析函数调用请求:
- 从 API 响应中提取函数名称和参数。
- 调用本地函数:
- 根据函数名称和参数执行预定义函数。
- 返回结果:
- 将函数执行结果返回给 AI 模型,生成最终响应。
- 示例代码:
if completion.choices[0].message.tool_calls: function_name = completion.choices[0].message.tool_calls[0].function.name arguments_string = completion.choices[0].message.tool_calls[0].function.arguments arguments = json.loads(arguments_string) # 创建一个函数映射表 function_mapper = { "get_current_time": get_current_time } # 获取函数实体 function = function_mapper[function_name] if arguments == {}: function_output = function() else: function_output = function(arguments) print(f"AI:{function_output}\n")
5. 完整代码示例
- 完整代码
#-*-coding:utf-8-*-
from openai import OpenAI
import json
from datetime import datetime
# 1. 外部工具函数调用定义
def get_current_time():
current_datetime = datetime.now()
formatted_time = current_datetime.strftime('%Y-%m-%d %H:%M:%S')
return f"现在是 {formatted_time}"
## 步骤2:创建 tools 数组,传递给LLM的知识库之一
functions = [
{
"type": "function",
"function": {
"name": "get_current_time",
"description": "当你想知道现在的时间时非常有用。",
}
},
]
# 步骤3:发起 function calling 请求
client = OpenAI(
api_key="your-api-key",
base_url="https://api.deepseek.com/"
)
def chat_completion_request(messages, tools):
print(f"正在像LLM发起API请求...")
completion = client.chat.completions.create(
model="deepseek-chat", # 此处以deepseek-chat为例
messages=messages,
tools=tools
)
print("返回对象:")
print(completion.choices[0].message.model_dump_json())
print("\n")
return completion
def main_loop():
print("欢迎使用智能助手!输入内容开始对话(输入 exit 退出)")
while True:
try:
# 获取用户输入
user_input = input("\n用户输入: ")
if user_input.lower() in ("exit", "quit"):
break
# 创建messages数组
messages = [
{"role": "system", "content": "",},
{"role": "user", "content": user_input}
]
completion = chat_completion_request(messages, functions)
if not completion:
continue
if completion.choices[0].message.tool_calls:
function_name = completion.choices[0].message.tool_calls[0].function.name
arguments_string = completion.choices[0].message.tool_calls[0].function.arguments
arguments = json.loads(arguments_string)
# 创建一个函数映射表
function_mapper = {
"get_current_time": get_current_time
}
# 获取函数实体
function = function_mapper[function_name]
if arguments == {}:
function_output = function()
else:
function_output = function(arguments)
print(f"AI:{function_output}\n")
else:
print(f"AI:{completion.choices[0].message.content}\n")
except KeyboardInterrupt:
print("\n再见!")
break
except Exception as e:
print(f"发生错误: {str(e)}")
if __name__ == "__main__":
main_loop()
- 案例演示
- 使用DeepSeek网页版
- 使用Function Calling版
- 使用DeepSeek网页版
6. 功能扩展
- 多函数支持:
- 定义多个函数,支持更复杂的任务。
- 错误处理:
- 处理 API 调用失败、函数执行错误等情况。
- 上下文记忆:
- 在多轮对话中保留上下文,提升交互体验。
7. 总结
- 项目成果:
- 使用最简单的外部时间调用函数,实现了一个支持 Function Calling 的智能助手。
- 能够根据用户输入调用预定义函数并返回结果。
- 未来改进方向:
- 支持更多函数和复杂任务。
- 优化性能和用户体验。
8. 参考资料
更多推荐
所有评论(0)