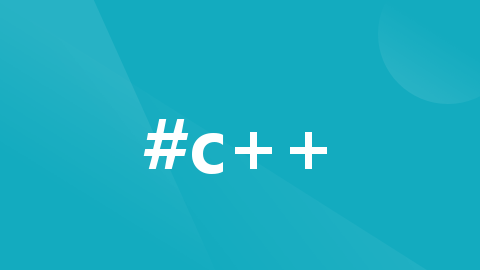
Day12 C++ 中 std::string::find()
·
#deepseek
C++ 中 std::string::find()
的用法详解:
一、函数作用
std::string::find()
用于在字符串中查找子字符串或字符的第一次出现位置。若未找到,返回 string::npos
。
二、函数原型
size_t find(const string& str, size_t pos = 0) const;
size_t find(const char* s, size_t pos = 0) const;
size_t find(char c, size_t pos = 0) const;
三、参数说明
参数 | 类型 | 说明 |
---|---|---|
str |
const string& |
要查找的子字符串 |
s |
const char* |
要查找的 C 风格字符串 |
c |
char |
要查找的单个字符 |
pos |
size_t |
开始查找的起始位置(默认为 0) |
四、返回值
-
返回找到的第一个匹配项的起始索引(从 0 开始)。
-
若未找到,返回
string::npos
(通常为size_t
类型的最大值)。
五、使用示例
1. 查找子字符串
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world! C++ is powerful.";
size_t pos = str.find("world");
if (pos != std::string::npos) {
std::cout << "Found at position: " << pos << std::endl; // 输出 7
} else {
std::cout << "Not found." << std::endl;
}
return 0;
}
2. 查找字符
size_t pos = str.find('C'); // 返回 13
3. 指定起始位置
// 从位置 10 开始查找逗号
size_t pos = str.find(',', 10); // 返回 npos(原字符串中逗号在位置 5)
六、常见问题及注意事项
1. 检查返回值
if (str.find("target") != std::string::npos) {
// 找到的逻辑
}
2. 区分大小写
// 查找 "hello"(全小写)
size_t pos = str.find("hello"); // 在 "Hello" 中找不到,返回 npos
3. 空字符串的特殊情况
size_t pos = str.find(""); // 始终返回 0
pos = str.find("", 5); // 返回 5(空字符串在任何位置匹配)
4. 查找所有出现的位置
std::string data = "ababaabba";
size_t pos = data.find("ab");
while (pos != std::string::npos) {
std::cout << "Found at: " << pos << std::endl; // 输出 0, 2, 5
pos = data.find("ab", pos + 1);
}
七、与其他查找函数对比
函数 | 作用 |
---|---|
rfind() |
从后向前查找 |
find_first_of() |
查找任何指定字符的首位置 |
find_last_of() |
查找任何指定字符的末位置 |
Leetcode
法一:
class Solution {
public:
bool repeatedSubstringPattern(string s) {
int n = s.length();
for(int i = 1; i<=n/2; i++){
if(n%i==0){
bool f = true;
for(int j = i; j < n; j++){
if(s[j] != s[j-i]){
f = false;
break;
}
}
if(f){
return true;
}
}
}
return false;
}
};
法二:
class Solution {
public:
bool repeatedSubstringPattern(string s) {
return (s+s).find(s,1) != s.size();
}
};
更多推荐
所有评论(0)