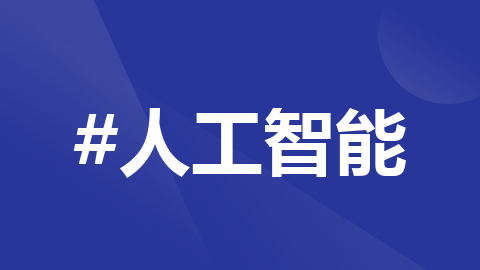
硅基流动API集成开发指南
·
一、环境准备
1.1 账号注册
访问硅基流动官网
完成开发者账号注册(注:新用户可领取试用资源)
邀请码 【nK76L7kR】
1.2 API密钥获取
- 登录控制台 -> 账户管理 -> API密钥
- 点击"新建密钥"生成
sk-xxxxxxxx
格式的访问凭证
# 建议将密钥存储在环境变量中
import os
os.environ['SILICONFLOW_API_KEY'] = 'sk-your-api-key-here'
二、API调用实践
2.1 文本生成接口
import requests
def chat_completion(prompt, model="deepseek-ai/DeepSeek-R1"):
url = "https://api.siliconflow.cn/v1/chat/completions"
headers = {
"Authorization": f"Bearer {os.getenv('SILICONFLOW_API_KEY')}",
"Content-Type": "application/json"
}
payload = {
"model": model,
"messages": [{"role": "user", "content": prompt}],
"temperature": 0.7
}
try:
response = requests.post(url, json=payload, headers=headers)
response.raise_for_status()
return response.json()['choices'][0]['message']['content']
except Exception as e:
print(f"API调用异常: {str(e)}")
return None
# 调用示例
result = chat_completion("用Python实现快速排序")
print(result)
2.2 多模态接口调用(以TTS为例)
def text_to_speech(text, voice="zh-CN-XiaoxiaoNeural"):
tts_url = "https://api.siliconflow.cn/v1/audio/speech"
headers = {
"Authorization": f"Bearer {os.getenv('SILICONFLOW_API_KEY')}",
"Content-Type": "application/json"
}
payload = {
"input": text,
"model": "siliconflow/tts-1",
"voice": voice
}
response = requests.post(tts_url, json=payload, headers=headers)
if response.status_code == 200:
with open("output.mp3", "wb") as f:
f.write(response.content)
return True
return False
三、私有化部署方案
3.1 客户端集成(以FastAPI为例)
from fastapi import FastAPI, HTTPException
app = FastAPI()
@app.post("/ai-assistant")
async def ai_assistant(prompt: str):
try:
response = chat_completion(prompt)
return {"status": "success", "data": response}
except Exception as e:
raise HTTPException(status_code=500, detail=str(e))
四、最佳实践建议
- 流量控制:通过令牌桶算法实现请求限流
- 错误处理:
# 重试机制示例
from tenacity import retry, stop_after_attempt, wait_exponential
@retry(stop=stop_after_attempt(3), wait=wait_exponential(multiplier=1, min=2, max=10))
def safe_api_call():
# API调用代码
- 安全规范:
- 敏感数据本地加密存储
- 使用HTTPS协议传输
- 定期轮换API密钥
五、常见问题排查
错误代码 | 解决方案 |
---|---|
401 Unauthorized | 检查API密钥有效性及Bearer前缀
2 |
429 Too Many Requests | 降低请求频率或升级服务套餐 |
503 Service Unavailable | 检查模型状态页或切换备用模型 |
说明文档注意事项:
- 避免包含推广性内容,专注技术实现细节
- 代码示例需包含完整错误处理
- 参数说明需标注取值范围和默认值
- 强调数据本地化处理的重要性(符合隐私保护要求)
更多推荐
所有评论(0)